Native SDK
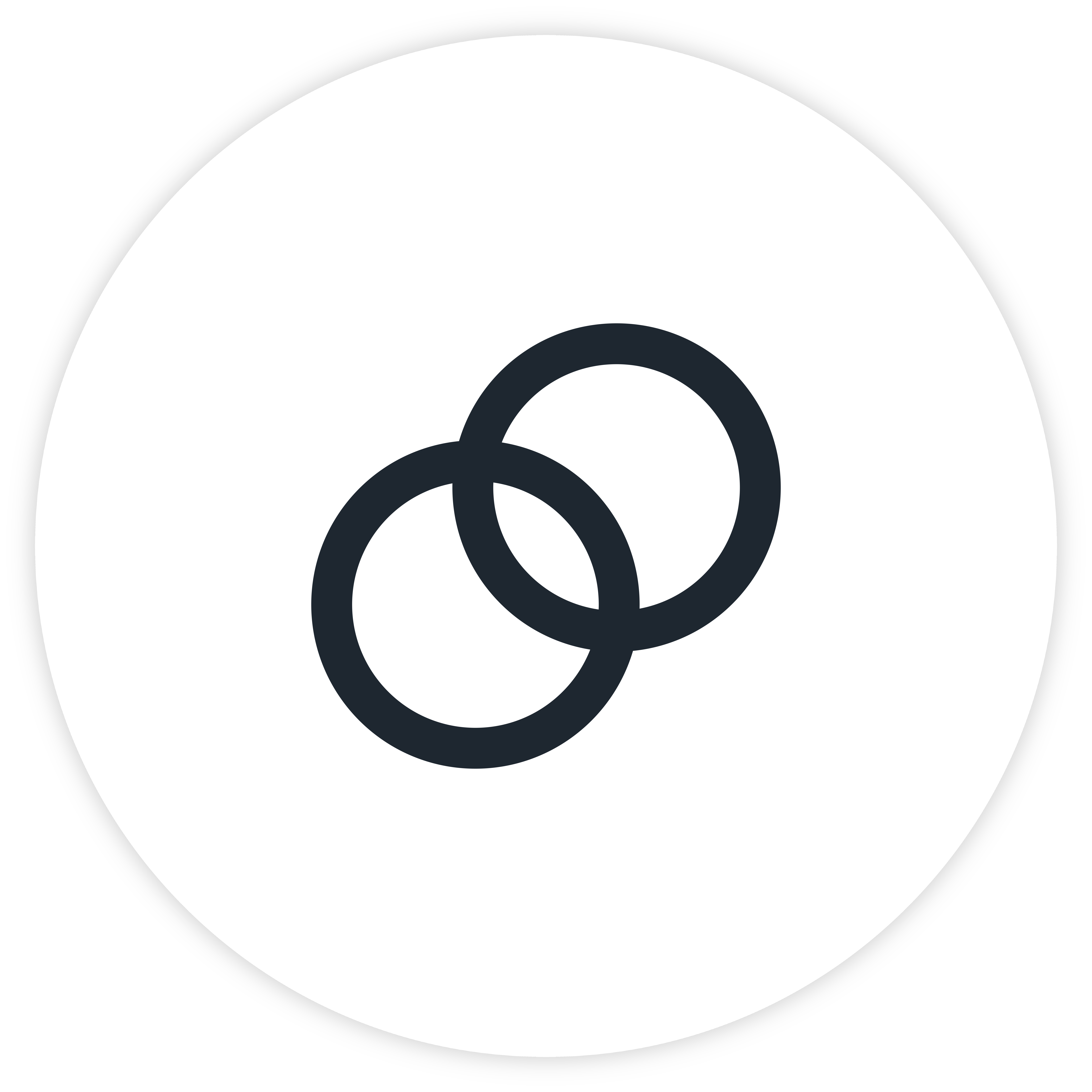
ethOS system wallet support
Adding support in native android for the system wallet is super easy, and there are two ways to do it:
1. CompletableFutures
If you are more comfortable with CompletableFutures, then you only need to add one thing to your project-level build.gradle file (To be able to download packages from jitpack):
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
maven { url 'https://jitpack.io' } // Add this
}
}
Then, in the app-level build.gradle you need to add 2 dependencies. The WalletSDK, and also Web3j, which the WalletSDK uses:
// Web3j needed for the WalletSDK
implementation 'org.web3j:core:4.8.8-android'
implementation 'com.github.EthereumPhone:WalletSDK:0.0.8'
Great! You now added the WalletSDK to your app. Now lets explore how to use it.
Testing
First, you can check whether the device has the system-level wallet with one if statement: getSystemService("wallet") != null
. Replace context here with your own context.
How to initialize the SDK:
// You just need to supply a context
val wallet = WalletSDK(context)
// You can also supply your own web3 rpc-url, like this:
val wallet = WalletSDK(
context = context,
web3RPC = "YOUR_URL"
)
How to sign a message:
// How to sign Message
wallet.signMessage(
message = "Message to sign"
).whenComplete { s, throwable ->
// Returns signed message
if (s == WalletSDK.DECLINE) {
println("Sign message has been declined")
} else {
println(s)
}
}
How to send a transaction:
// How to send send Transactions
wallet.sendTransaction(
to = "0x3a4e6ed8b0f02bfbfaa3c6506af2db939ea5798c", // mhaas.eth
value = "1000000000000000000", // 1 eth in wei
data = ""
).whenComplete {s, throwable ->
// Returns tx-id
if (s == WalletSDK.DECLINE) {
println("Send transaction has been declined")
} else {
println(s)
}
}
And that’s it for the CompletableFutures part. You should now be able to reference the system wallet to do transactions within your app!
If there are any other questions please feel free to reach out in our discord, or message me on telegram at @mhaas_eth
2. Coroutines
To add the WalletSDK to your app, make the same changes to the project-level build.gradle as in the CompletableFutures part above, & in the app-level build.gradle, add this:
// Web3j needed for the WalletSDK
implementation 'org.web3j:core:4.8.8-android'
implementation 'com.github.EthereumPhone:WalletSDK:0.0.10'
You can check whether the system-wallet is on the device by checking getSystemService("wallet") != null
.
How to initialize the SDK:
// You just need to supply a context
val wallet = WalletSDK(context)
// You can also supply your own web3j instance, like this:
val web3j = Web3j.build(HttpService("https://rpc.ankr.com/eth"))
val wallet = WalletSDK(
context = context,
web3RPC = web3j
)
How to sign a message:
// How to sign Message
CoroutineScope(Dispatchers.IO).launch {
val result = wallet.signMessage(
message = "Message to sign"
)
println(result)
}
How to send a transaction:
CoroutineScope(Dispatchers.IO).launch {
val result = wallet.sendTransaction(
to = "0x3a4e6ed8b0f02bfbfaa3c6506af2db939ea5798c", // mhaas.eth
value = "1000000000000000000", // 1 eth in wei
data = "")
println(result)
}
That’s all you should need to know for Coroutines. You should now be able to reference the system wallet to do transactions within your app!
If there are any other questions please feel free to reach out in our discord, or message me on telegram at @mhaas_eth
.
Updated 2 months ago